# Let’s Display the Second Screen
On this page, I’ll explain how to display the second screen. You can also try it out with the sample included in the Plugin.
:::caution Dual-screen display does not function in Unity Editor. Please Build and run your app to confirm. :::
## Prerequisites - Setup your Spatial Reality Display, if not, see: Setup [Spatial Reality Display](/Products/Developer-Spatial-Reality-display/en/develop/Setup/SetupSRDisplay.html), [Setup Spatial Reality Display Settings](/Products/Developer-Spatial-Reality-display/en/develop/Setup/SetupSRRuntime.html) - Set up your Unity project installed SRDisplay UnityPlugin, if not, see: [Set up for Unity](/Products/Developer-Spatial-Reality-display/en/develop/Unity/Setup.html)
## How to Display the Second Screen Refer to [Create your simple app "Hello cube!"](/Products/Developer-Spatial-Reality-display/en/develop/Unity/HelloCubeApp.html) to place the SRDisplayManager Prefab. To display the second screen, first call Init2DView() on the SRDisplayManager. Then, obtain SRD2DView. ```cs if (_srdManager.Init2DView()) { _srd2DView = _srdManager.SRD2DView; } ``` Use Show(true) to display the second screen. ```cs _srd2DView.Show(true); ``` 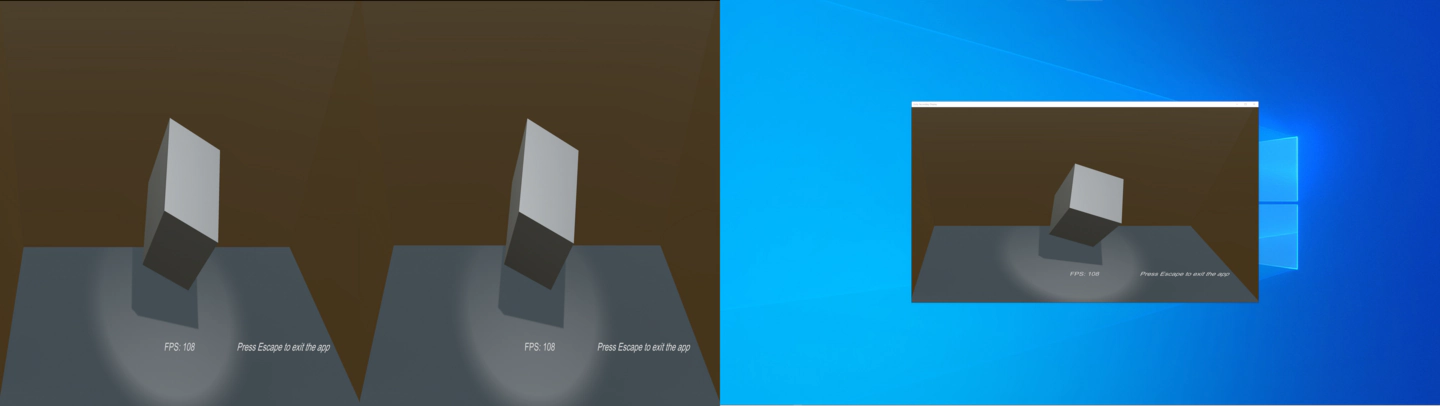{.img83} To hide the second screen, set it to false. ```cs _srd2DView.Show(false); ``` 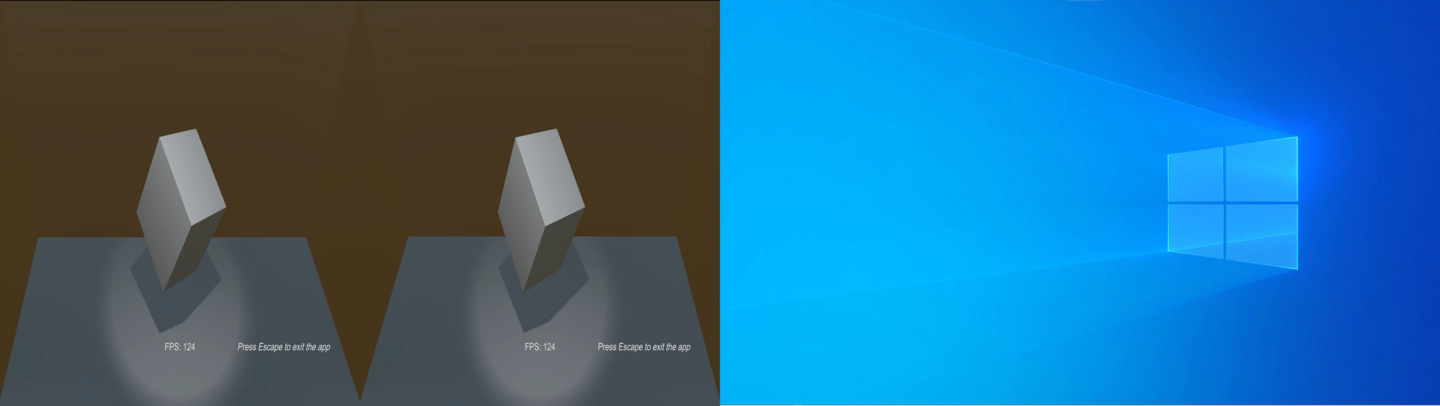{.img83} In the sample, the F5 key is assigned for toggling display/hide of the second screen.
## How to display the Second Screen in Full Screen You can display the second screen in full screen. ```cs _srd2DView.SetFullScreen(true); ``` 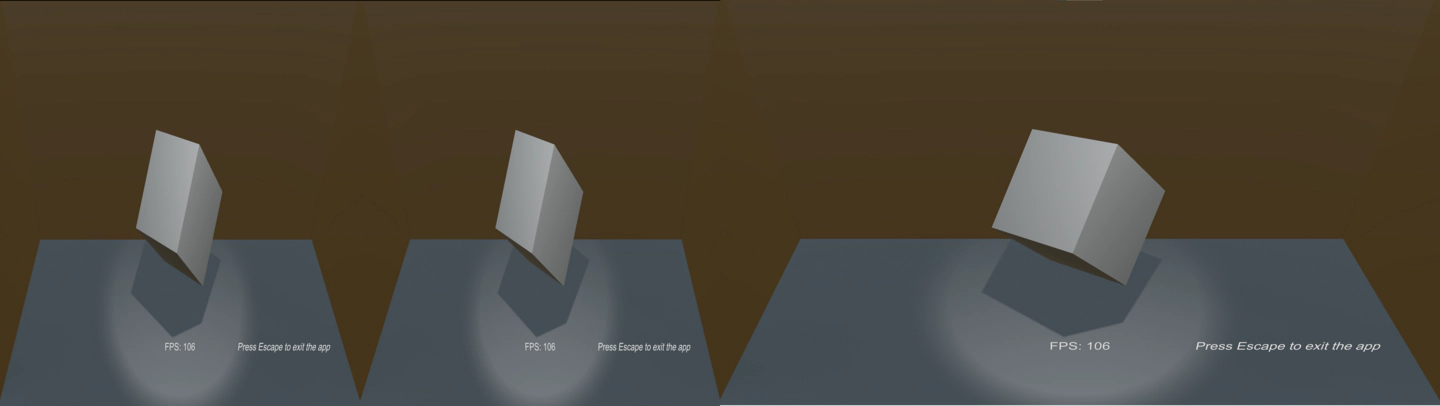{.img83} To revert from full screen, use: ```cs _srd2DView.SetFullScreen(false); ``` In the sample, you can check this using the F6 key (toggle full screen).
## How to Set Images for the Second Screen You can configure images for display, including left-eye images, right-eye images, and mixed images on the Spatial Reality Display. Set the left-eye image. ```cs _srd2DView.SetSourceTexture(SRDTextureType.LeftEye); ``` Set the right-eye image. ```cs _srd2DView.SetSourceTexture(SRDTextureType.RightEye); ``` Set the mixed image. ```cs _srd2DView.SetSourceTexture(SRDTextureType.SideBySide); ``` ELF-SR2: {.img83} ELF-SR1: 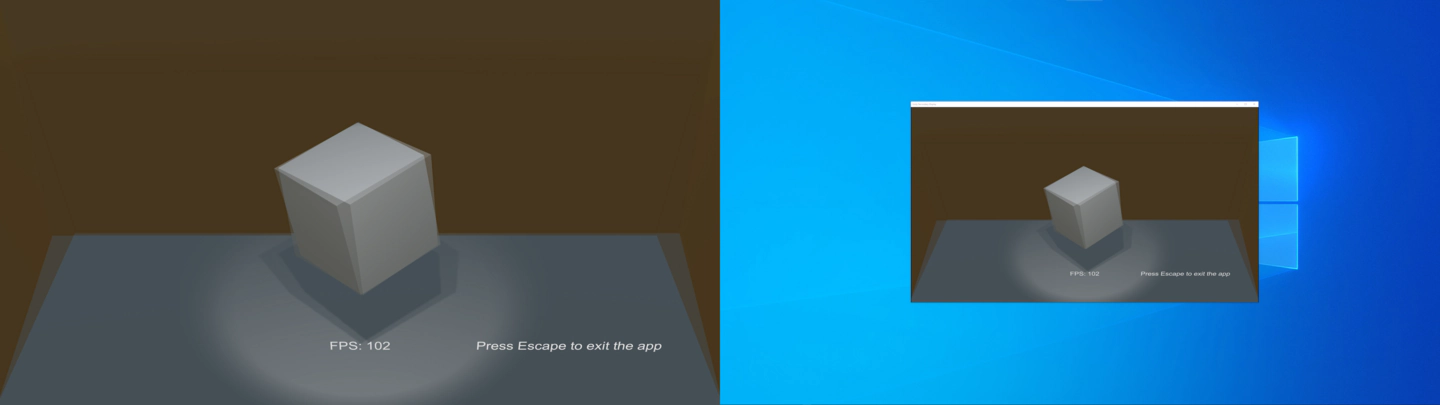{.img83} In the sample, you can switch between the texture types using the F7 key. **Set a custom texture.** {.newlabel-2024-10-29} Starting from SDK 2.4.0, you can also display the texture of your choice (CustomTexture)。 To display a custom texture, call `SetSourceTexture()` with the `SRDTextureType.Custom` parameter, and assign the texture you want to display to `CustomTexture`. For example, if you want to place a camera in the scene and display the image from that camera on the Second Screen, your implementation might look like this: ```cs public void ShowCameraImage(Camera sourceCamera) { _srd2DView.SetSourceTexture(SRD2DView.SRDTextureType.Custom); _srd2DView.CustomTexture = sourceCamera.activeTexture; } ``` {.img83} See Sample 6 for an example of how to use CustomTexture.
:::caution Dual-screen display does not function in Unity Editor. Please Build and run your app to confirm. :::
## Prerequisites - Setup your Spatial Reality Display, if not, see: Setup [Spatial Reality Display](/Products/Developer-Spatial-Reality-display/en/develop/Setup/SetupSRDisplay.html), [Setup Spatial Reality Display Settings](/Products/Developer-Spatial-Reality-display/en/develop/Setup/SetupSRRuntime.html) - Set up your Unity project installed SRDisplay UnityPlugin, if not, see: [Set up for Unity](/Products/Developer-Spatial-Reality-display/en/develop/Unity/Setup.html)
## How to Display the Second Screen Refer to [Create your simple app "Hello cube!"](/Products/Developer-Spatial-Reality-display/en/develop/Unity/HelloCubeApp.html) to place the SRDisplayManager Prefab. To display the second screen, first call Init2DView() on the SRDisplayManager. Then, obtain SRD2DView. ```cs if (_srdManager.Init2DView()) { _srd2DView = _srdManager.SRD2DView; } ``` Use Show(true) to display the second screen. ```cs _srd2DView.Show(true); ``` 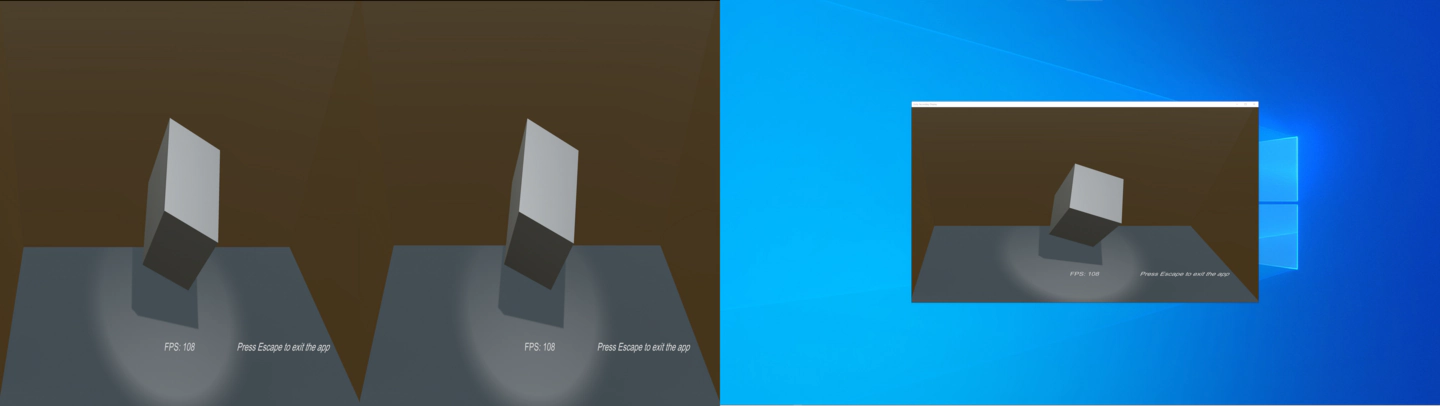{.img83} To hide the second screen, set it to false. ```cs _srd2DView.Show(false); ``` 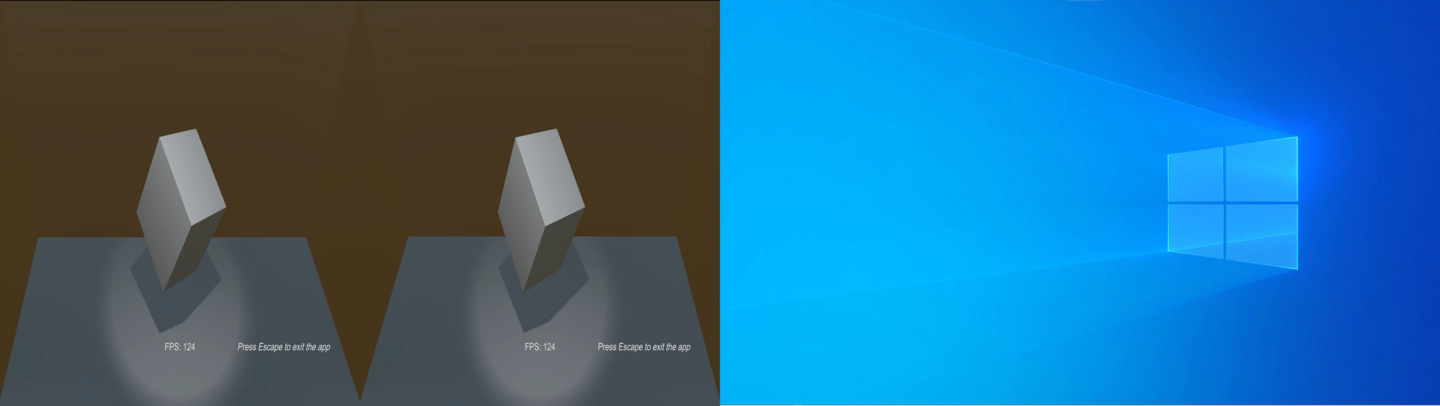{.img83} In the sample, the F5 key is assigned for toggling display/hide of the second screen.
## How to display the Second Screen in Full Screen You can display the second screen in full screen. ```cs _srd2DView.SetFullScreen(true); ``` 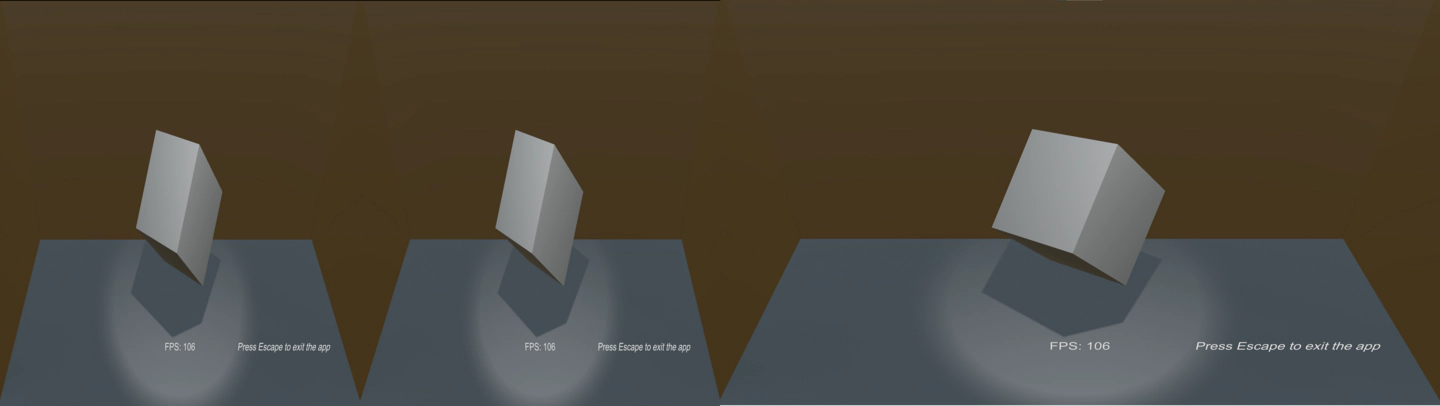{.img83} To revert from full screen, use: ```cs _srd2DView.SetFullScreen(false); ``` In the sample, you can check this using the F6 key (toggle full screen).
## How to Set Images for the Second Screen You can configure images for display, including left-eye images, right-eye images, and mixed images on the Spatial Reality Display. Set the left-eye image. ```cs _srd2DView.SetSourceTexture(SRDTextureType.LeftEye); ``` Set the right-eye image. ```cs _srd2DView.SetSourceTexture(SRDTextureType.RightEye); ``` Set the mixed image. ```cs _srd2DView.SetSourceTexture(SRDTextureType.SideBySide); ``` ELF-SR2: {.img83} ELF-SR1: 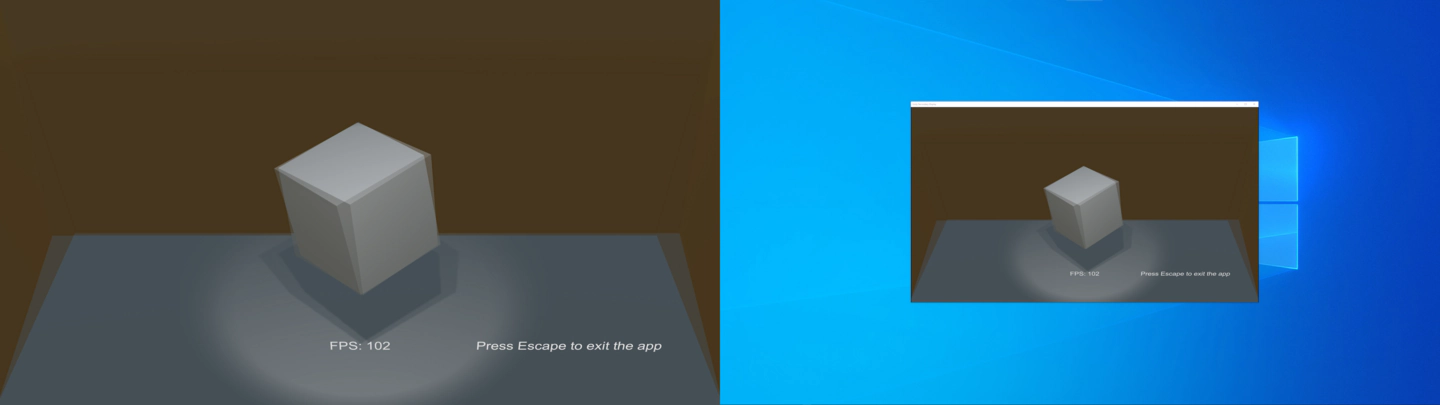{.img83} In the sample, you can switch between the texture types using the F7 key. **Set a custom texture.** {.newlabel-2024-10-29} Starting from SDK 2.4.0, you can also display the texture of your choice (CustomTexture)。 To display a custom texture, call `SetSourceTexture()` with the `SRDTextureType.Custom` parameter, and assign the texture you want to display to `CustomTexture`. For example, if you want to place a camera in the scene and display the image from that camera on the Second Screen, your implementation might look like this: ```cs public void ShowCameraImage(Camera sourceCamera) { _srd2DView.SetSourceTexture(SRD2DView.SRDTextureType.Custom); _srd2DView.CustomTexture = sourceCamera.activeTexture; } ``` {.img83} See Sample 6 for an example of how to use CustomTexture.