# Let's create a GUI on the second screen
With the release of SDK ver.2.4.0, developers are now able to set any texture of their choice (CustomTexture) to be displayed to the second screen (SRD2DView), as well as to place buttons or other interactible GUI elements. \
In this article, we will introduce how to create a simple application making use of these new features.
:::note
Please see the page below for details about the basic usage of the second screen feature. \
[Let's Display the Second Screen.](/Products/Developer-Spatial-Reality-display/en/develop/Unity/lets-display-the-second-screen/)
:::
## Starting Up
:::note
For this article, we will use Unity 2022.3 with a URP project.
:::
Let's start by creating a new Unity Project and by importing the srdisplay-unity-plugin.unitypackage ver.2.4.0. \
Delete all objects in the default SampleScene except the Directional Light, and add an SRDisplayManager and an SRDisplayBox to the scene.
Next, add a new 3D Object to the center of the SRDisplayBox and attach the `Floating Object` script to it. \
Finally, create the following `AppController` script and attach it to a new empty GameObject so that the `SR2DView` appears right when the app starts.
```csharp
using UnityEngine;
using SRD.Core;
using SRD.Utils;
public class AppController : MonoBehaviour
{
private SRDManager _srdManager;
private SRD2DView _srd2DView;
void Start()
{
_srdManager = SRDSceneEnvironment.GetSRDManager();
if (_srdManager.Init2DView())
{
_srd2DView = _srdManager.SRD2DView;
}
}
void Update()
{
if (Input.GetKeyDown(KeyCode.Escape))
{
Application.Quit();
}
}
}
```
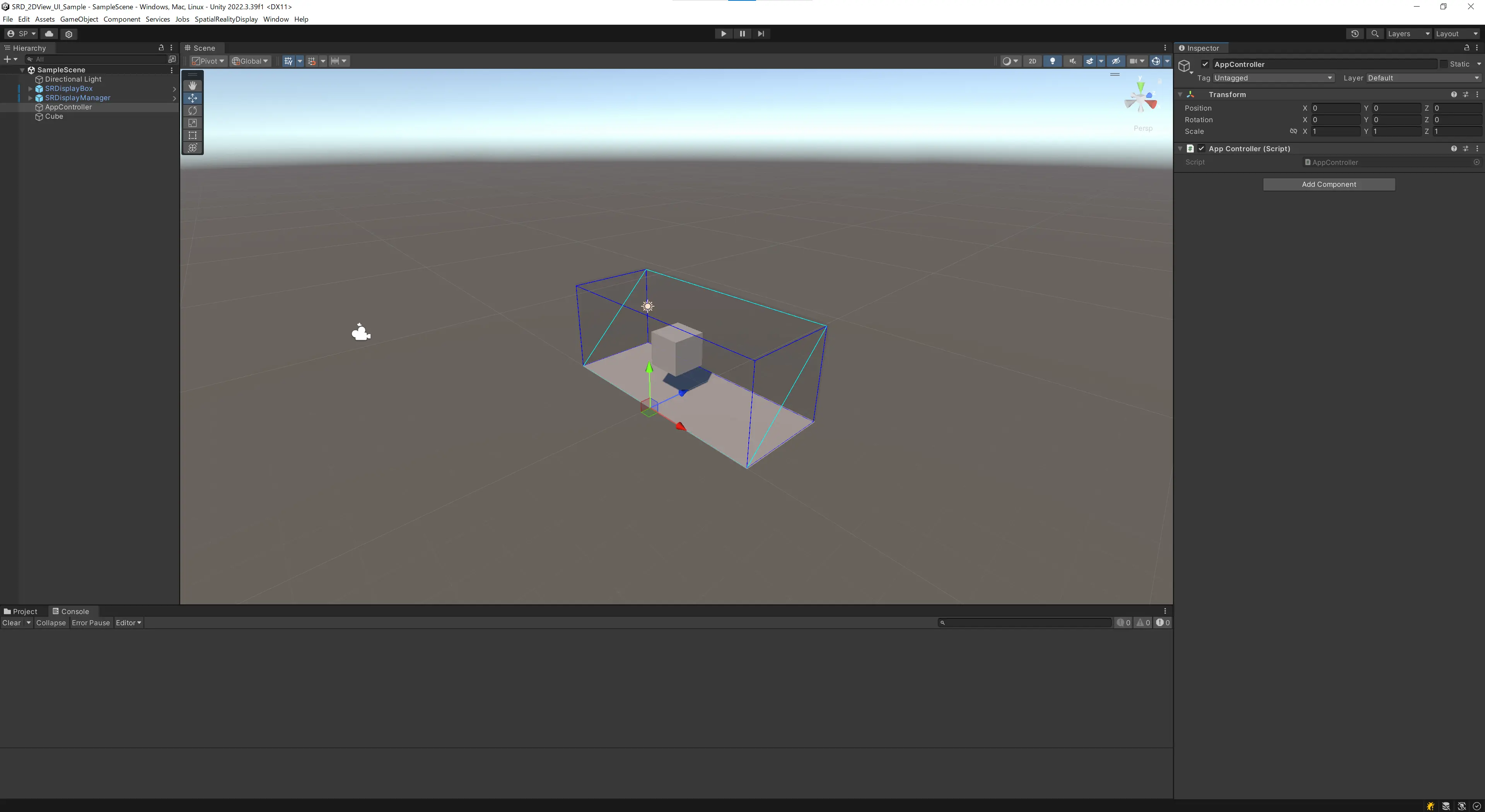{.img100}
## CustomTexture
First, let's use CustomTexture to display the scene from a different perspective on the second screen.
1. Create a new RenderTexture in the Assets folder.
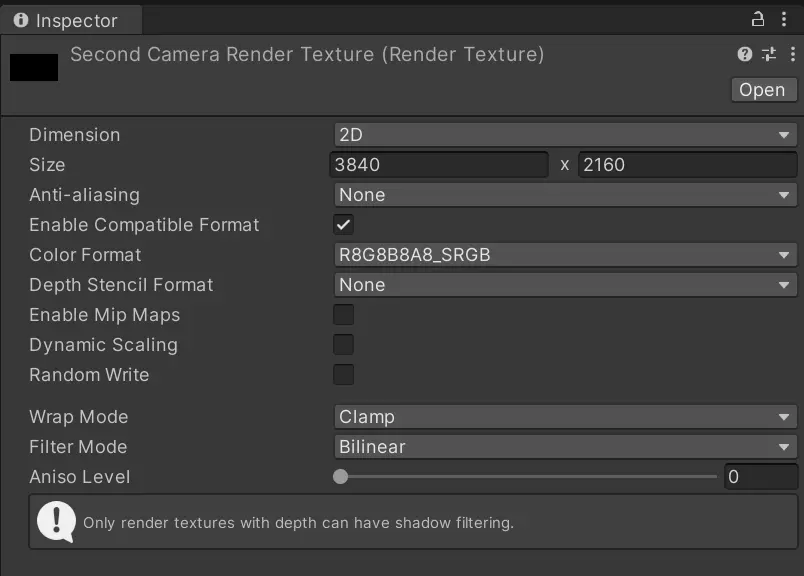
2. Place a new Camera object, `SecondCamera`, in the scene in the desired location and assign the RenderTexture you just created to its Output Texture.
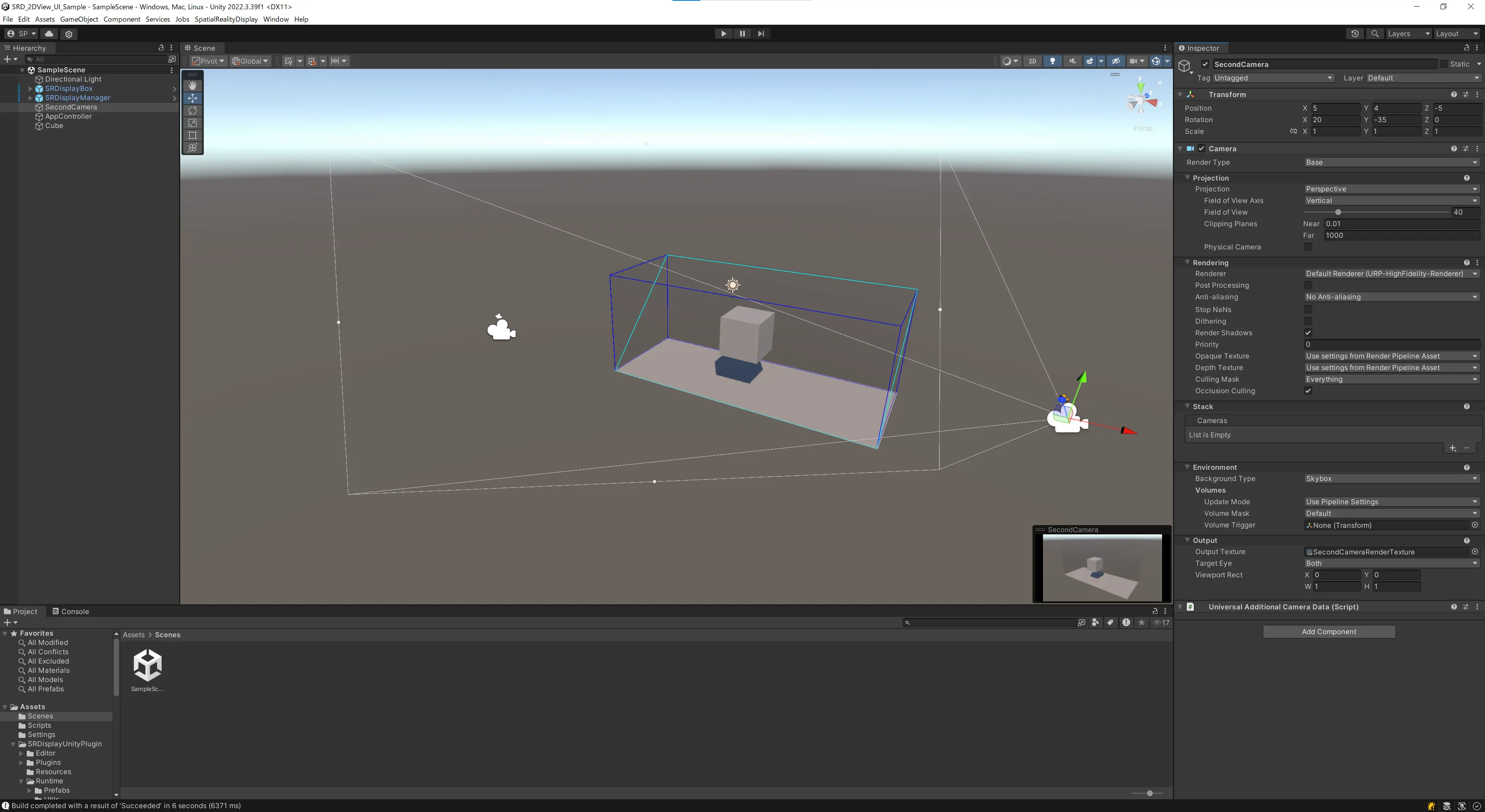{.img100}
3. Make the following changes to AppController.
4. In the Inspector, assign the SecondCamera object to the AppController's `Second Camera`.
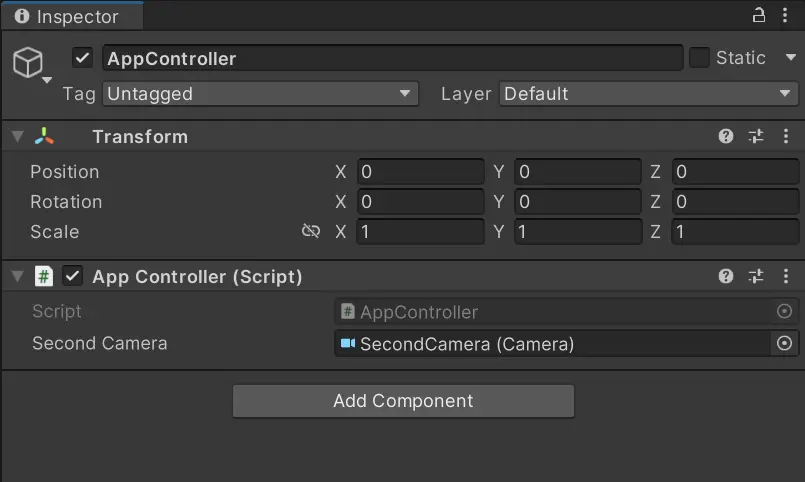
Build the app and run the exe. The image of the `Second Camera` will be displayed on the second screen.
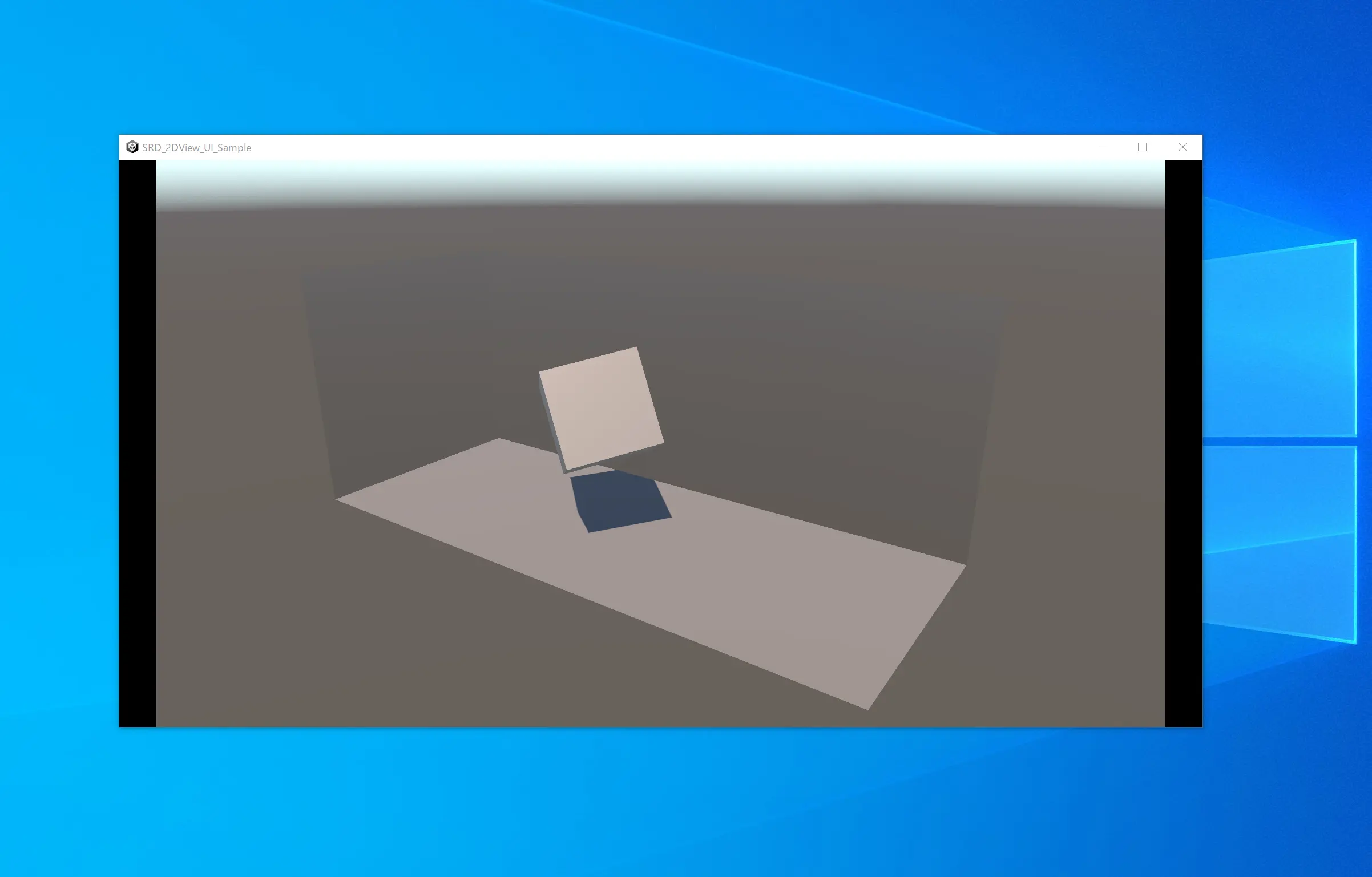{.img100}
## GUI
Next, let's create a button to switch the image displayed on the second screen.
In normal Unity applications, the Canvas feature is used to create a GUI. \
Internally, `SR2DView` also uses a Canvas. When the `Init` function is called, a `Screen Space - Overlay` mode Canvas set to display on the second screen is automatically generated. Then an image set to display the current `SourceTexture` is placed on it so as to fill the whole screen. \
To display a button on the second screen, we also need to use a Canvas, but it requires some tweaking.
1. Create a new Screen Space Canvas. The Sort Order must be set to **1 or more** so that it appears on top of the Canvas inside `SR2DView`. Moreover, since the display on which `SR2DView` is displayed may differ depending on the PC environment, the following script needs to be attached to the Canvas to set the correct Target Display at runtime.
```csharp
using SRD.Core;
using UnityEngine;
[RequireComponent(typeof(Canvas))]
public class SecondDisplayCanvas : MonoBehaviour
{
void Start()
{
var canvas = GetComponent<Canvas>();
canvas.targetDisplay = SRD2DView.DisplayIndex;
}
}
```
2. Add a new Button object to the Canvas.
3. Add the following method to the AppController and link it to the Button's OnClick() event.
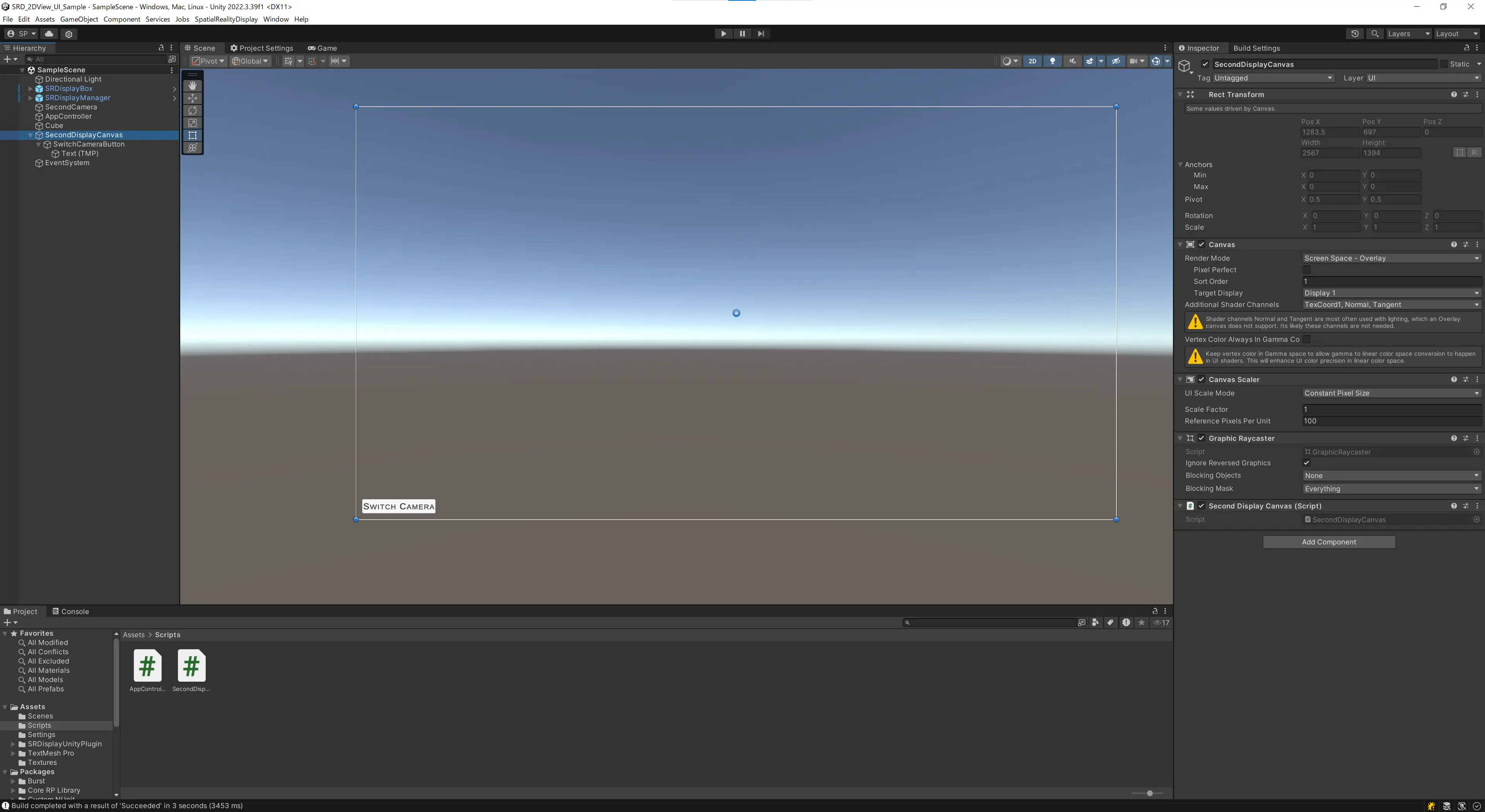{.img100}
Build the app again and run the exe. \
The "SWITCH CAMERA" button will appear in the bottom left of the second screen. Clicking it will switch between the SRDisplayManager's `LeftEye` image and `SecondCamera` image.
:::note
If you would like to know more about how to create a GUI for the second screen, please refer to the newly added sample in Spatial Reality Display Plugin for Unity ver.2.4.0. \
[6 - SRDisplay2DViewUISample](/Products/Developer-Spatial-Reality-display/en/develop/Unity/Samples.html#link_8)
:::
Prepare a variable for `SecondCamera`.
```csharp
[SerializeField]
private Camera _secondCamera;
```
Set the SecondCamera's TargetTexture to the SR2DView's CustomTexture at Start().
```csharp
void Start()
{
_srdManager = SRDSceneEnvironment.GetSRDManager();
if (_srdManager.Init2DView())
{
_srd2DView = _srdManager.SRD2DView;
if (_secondCamera != null)
{
_srd2DView.CustomTexture = _secondCamera.targetTexture;
}
}
}
```
```csharp
public void SwitchCamera()
{
if (_srd2DView.SourceTexture != SRD2DView.SRDTextureType.Custom)
{
_srd2DView.SetSourceTexture(SRD2DView.SRDTextureType.Custom);
}
else
{
_srd2DView.SetSourceTexture(SRD2DView.SRDTextureType.LeftEye);
}
}
```
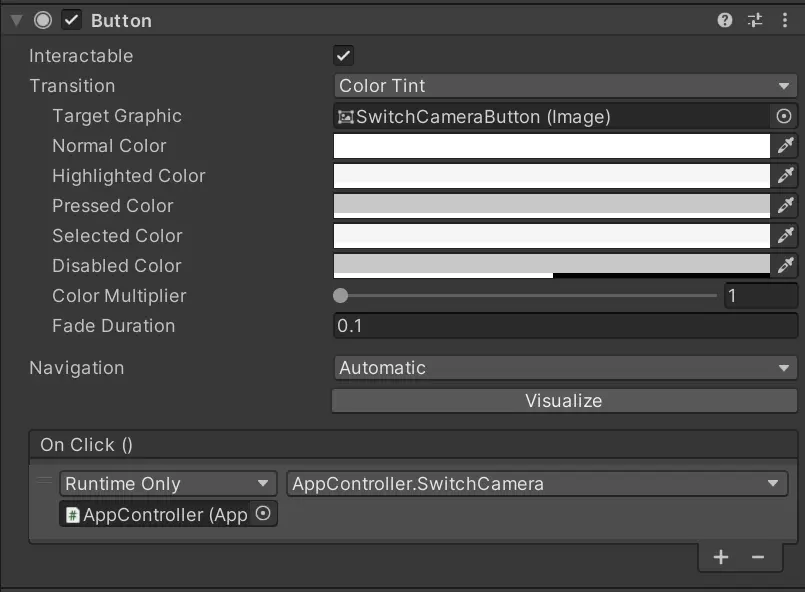{.img100}
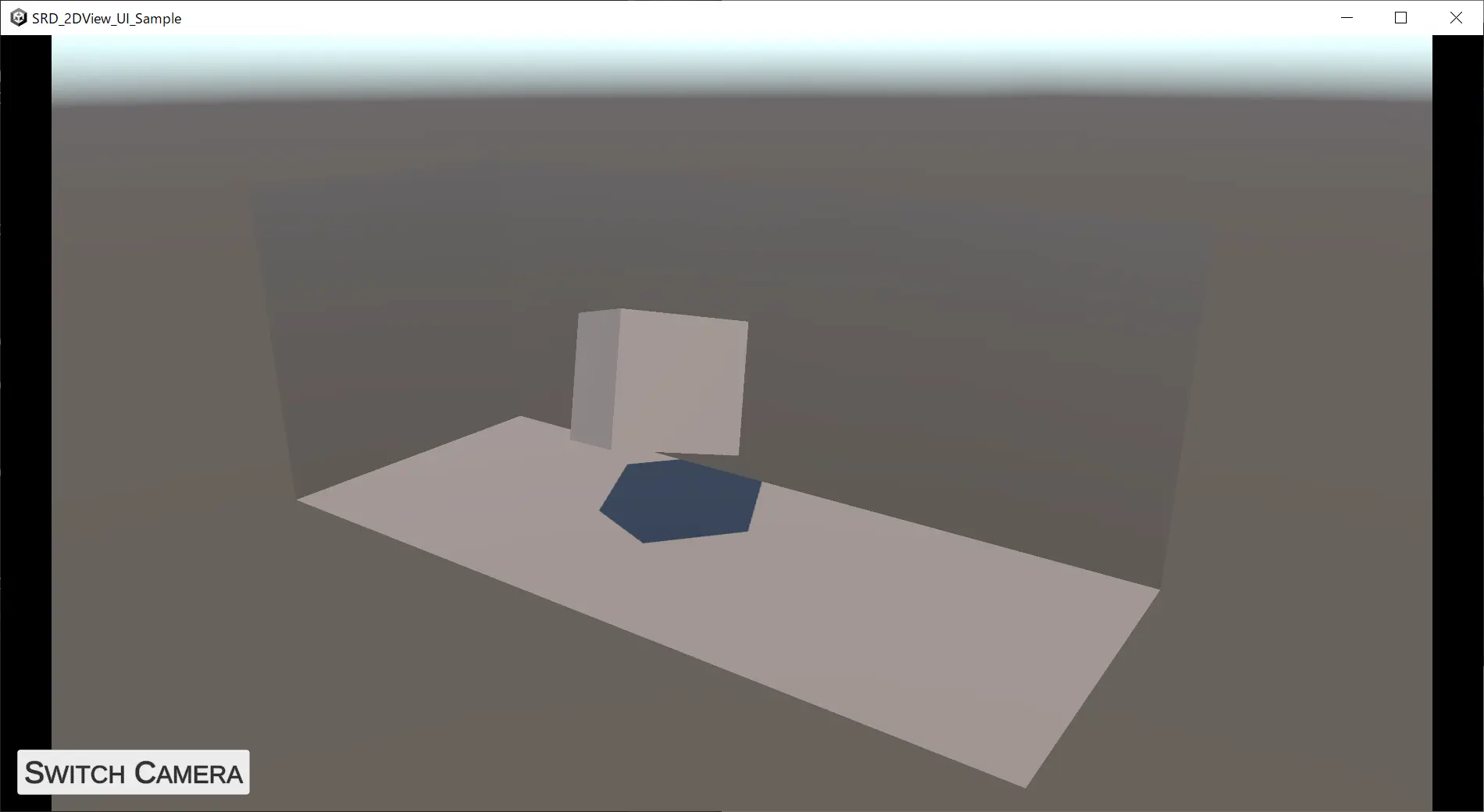{.img100}
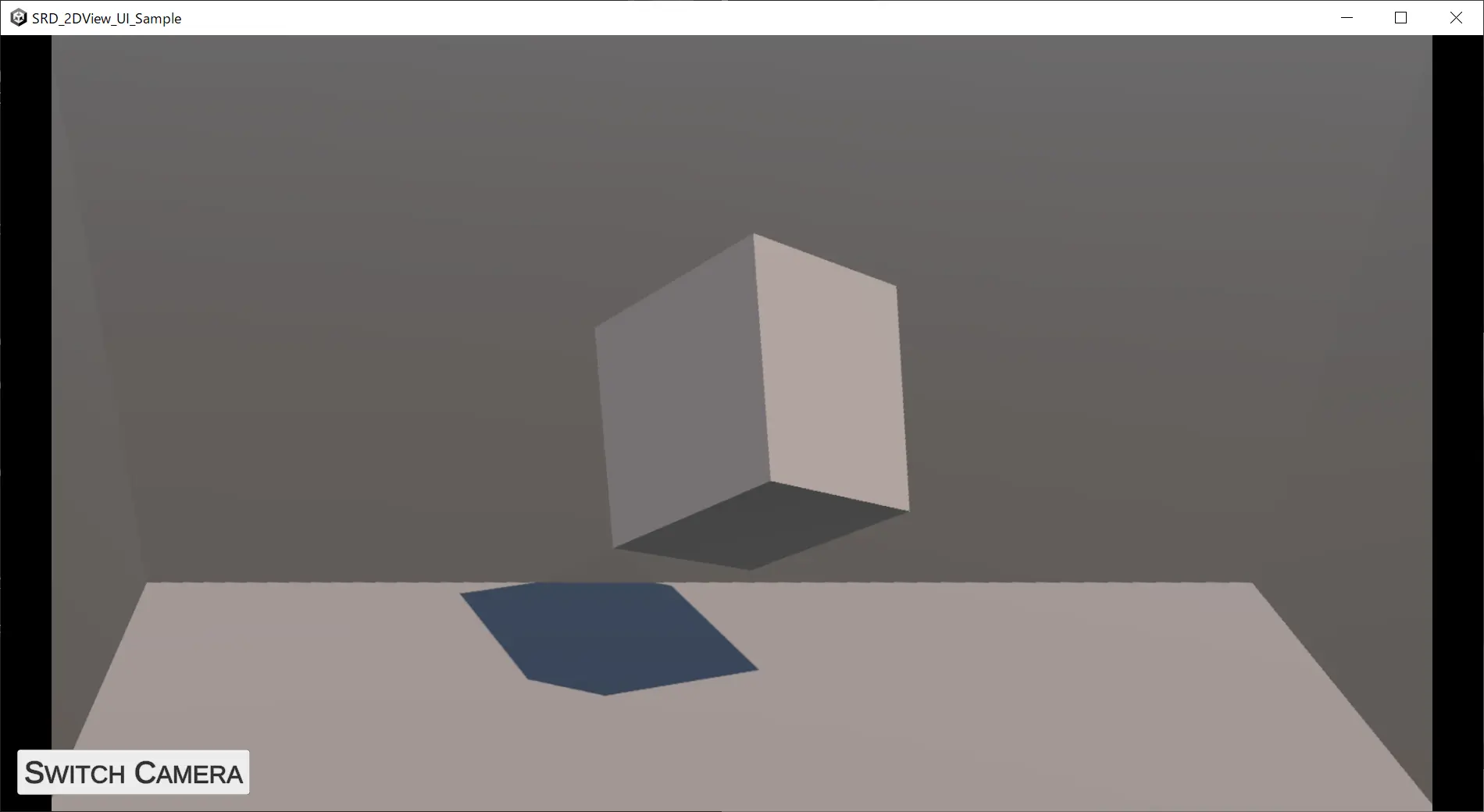{.img100}